Python Quiz 01
Do you know all the frequently used ticks in Python?
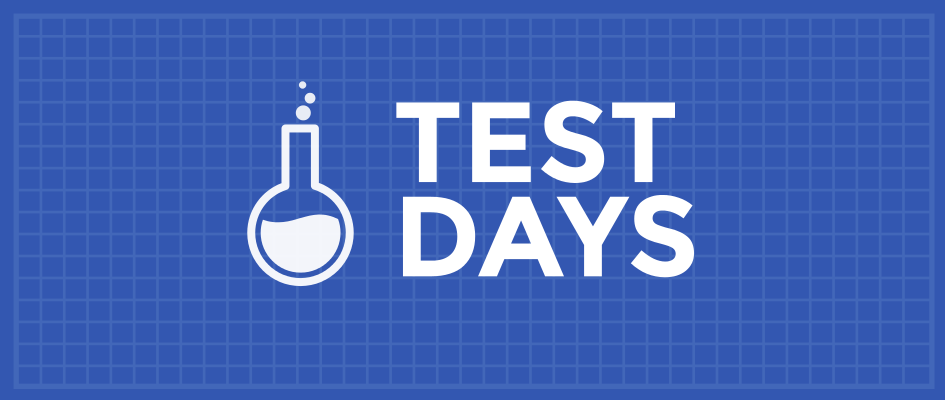
Bring out a white paper and write all the answers to the quiz on the paper. See if you can finish all of these answers.
How do you create a array which contains 2 - 10 in one-liner?
CLICK ME
# using list comprehension [i for i in range(2, 11)] # Or list(range(2, 11))
How do you randomly select 3 numbers from an integer array “‘A”’?
import random random.sample(A, 3)
How do you create a array of length 13 that for index > 4 its element is any random lowercase vowel(‘a’, ‘e’, ‘i’, ‘o’, ‘u’) but for index in [0, 4], its element is the square root value of the index?
import random # random.sample's return type is a list [i**0.5 if i < 5 else random.sample('aeiou', 1)[0] for i in range(13)]
How do you you heap sort an array A? How do you add a new value into a heap? How do you pop the root value from a heap? How do you add a new number to a min heap with a fixed length?
import heapq heapq.heapify(A) heapq.heappush(A, a) b = heapq.heappop(A) b = heapq.heappushpop(A, a)
How do you find all the permutations of a string
s
?import itertools itertools.permutations(s)
How do you combine two same-length arrays A and B as a pair array?
zip(A, B) # if A and B is not same length, what's the result?
Given a key “WTF” and a dictionary d, how do you find the value of the key and if the key doesn’t exist in the dict, can you return “” instead?(one-liner)
d.get("WTF", "")
How do you replace all the “is” to “si” in string “isawakiss”?
"isawakiss".replace("is", "si")
How do you replace all the vowels in a string to
'_'
?(regex)import re re.sub("[aeiou]", "_", s)
lowercase and uppercase of the string
s
?s.lower() s.upper() # print the alphabet import string string.ascii_letters string.ascii_lowercase
convert all the elements in string array
S
into the form specified in question 9, and combine the result string array into one huge string?''.join([re.sub('[aeiou]', '_', s) for s in S])
how do you convert base-2 binary number string to int?
int("10001", 2)
how do you find the binary expression of a 10-base number?
bin(23)[2:]
Do you know how to do string formatting in python? For example, given a list A, can you print something like “hey, look. This is a list: ####?” “####” represents the contents in the list.
"hey, look. This is a list: %s" % A "hey, look. This is a number: %d" % 23 "hey, look. This is a string: %s" % "WTF" "hey, look. This is a float number: %f " % 3.14
What is the output of the following program?
names = "{1}, {2} and {0}".format('John', 'Bill', 'Sean') print(names)
Bill, Sean and John
How to print the clock time in this format “XX:XX:XX” given the total seconds
s
? (one-liner)"{:02d}:{:02d}:{:02d}".format(secs // 3600, *divmod(secs % 3600, 60)) # print the clock time import time time.strttime('%H : %M : %S') time.strttime('%I : %M : %S %p')
What is the built-in functions to convert between ASCII numbers and characters?
ord('s') chr(65)
How do you determine if a character is a digit or not, is a letter or not?
's'.isdigit() 's'.isalpha()
Share this post
Twitter
Google+
Facebook
Reddit
LinkedIn
StumbleUpon
Pinterest
Email